Obfuscating Blacklisted Words In WordPress With ROT13
Countless algorithms for encrypting data exist in computer science. One of the lesser known and less common encryptions is ROT13, a derivative of the Caesar cypher encryption technique.
In this tutorial, we’ll learn about ROT13 encryption and how it works. We’ll see how text (or strings) can be programmatically encoded in ROT13 using PHP. Finally, we’ll code a WordPress plugin that scans a post for blacklisted words and replaces any in ROT13 encryption.
If you own a blog on which multiple authors or certain group of people have the privilege of publishing posts, then a plugin that encrypts or totally removes inappropriate words might come in handy
Before we begin let’s clear up something about ROT13. It should never be used to encrypt sensitive data. While it is considered an encryption technique, it is the “Hello World” example of encryption. It can be broken extremely easily and thus is never used on data which is encrypted for security reasons. Since our goal isn’t to protect data it is to hide profanity, it will do just fine for our example.
Introduction
ROT13 (short for “rotate by 13 places,” sometimes abbreviated as ROT-13) is a simple encryption technique for English that replaces each letter with the one 13 places forward or back along the alphabet. So, A becomes N, B becomes O and so on up to M, which becomes Z. Then, the sequence continues at the beginning of the alphabet: N becomes A, O becomes B and so on up to Z, which becomes M.
A major advantage of ROT13 over other rot(N)
techniques (where “N” is an integer that denotes the number of places down the alphabet in a Caesar cypher encryption) is that it is “self-inverse,” meaning that the same algorithm is applied to encrypt and decrypt data.
Below is a ROT13 table for easy reference.
| A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
--------------------------------------------------------------------------------------------------------
| N | O | P | Q | R | S | T | U | V | W | X | Y | Z | A | B | C | D | E | F | G | H | I | J | K | L | M |
If we encrypted the domain smashingmagazine.com
in ROT13, the result would be fznfuvatzntnmvar.pbz
, and the sentence “Why did the chicken cross the road?” would become “Jul qvq gur puvpxra pebff gur ebnq?”
Note that only letters in the alphabet are affected by ROT13. Numbers, symbols, white space and all other characters are left unchanged.
Transforming Strings To ROT13 In PHP
PHP includes a function, str_rot13()
, for converting a string to its ROT13-encoded value. To encode text in ROT13 using this function, pass the text as an argument to the function.
<?php
echo str_rot13('smashingmagazine.com'); // fznfuvatzntnmvar.pbz
echo str_rot13('The best web design and development blog'); // Gur orfg jro qrfvta naq qrirybczrag oybt
Using ROT13 In WordPress
Armed with this knowledge, I thought of ways it might be handy in WordPress. I ended up creating a plugin that encodes blacklisted words found in posts using ROT13.
The plugin consists of a textearea
field (located in the plugin’s settings page) in which you input blacklisted words, which are then saved to the database for later reuse in WordPress posts.
Without further fussing, let’s start coding the plugin.
Setting Up The Plugin
First, include the plugin’s file header.
<?php
/*
Plugin Name: Rot13 Words Blacklist
Plugin URI: https://smashingmagazine.com/
Description: A simple plugin that detects and encrypts blacklisted words in ROT13
Version: 1.0
Author: Agbonghama Collins
Author URI: https://w3guy.com
Text Domain: rot13
Domain Path: /lang/
License: GPL2
*/
As mentioned, the plugin will have a settings page with a textarea
field that collects and saves blacklisted words to WordPress’ database (specifically the options
table).
Below is a screenshot of what the plugin’s settings (or admin) page will look like.
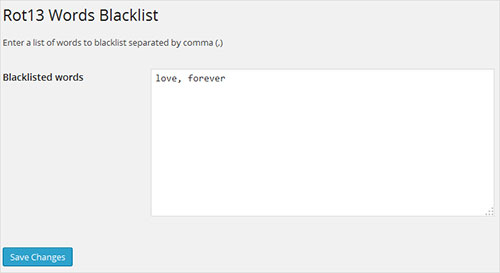
Now that we know what the options page will look like, let’s build it using WordPress’ Settings API
Building The Settings Page
First, we create a submenu item in the main “Settings” menu by using add_options_page()
, with its parent function hooked to admin_menu
action.
add_action( 'admin_menu', 'rot13_plugin_menu' );
/**
* Add submenu to main Settings menu
*/
function rot13_plugin_menu() {
add_options_page(
__( 'Rot13 Blacklisted Words', 'rot13' ),
__( 'Rot13 Blacklisted Words', 'rot13' ),
'manage_options',
'rot13-words-blacklist',
'rot13_plugin_settings_page'
);
}
The fifth parameter of add_options_page()
is the function’s name (rot13_plugin_settings_page
), which is called to output the contents of the page.
Below is the code for rot13_plugin_settings_page()
.
/**
* Output the contents of the settings page.
*/
function rot13_plugin_settings_page() {
echo '<div class="wrap">';
echo '<h2>', __( 'Rot13 Blacklisted Words', 'rot13' ), '</h2>';
echo '<form action="options.php" method="post">';
do_settings_sections( 'rot13-words-blacklist' );
settings_fields( 'rot13_settings_group' );
submit_button();
}
Next, we add a new section to the “Settings” page with add_settings_section()
. The textarea
field we mentioned earlier will be added to this section with add_settings_field()
. Finally, the settings are registered with register_setting()
.
Below is the code for add_settings_section()
, add_settings_field()
and register_setting()
.
// Add the section
add_settings_section(
'rot13_setting_section',
’,
'rot13_setting_section_callback_function',
'rot13-words-blacklist'
);
// Add the textarea field to the section.
add_settings_field(
'blacklisted_words',
__( 'Blacklisted words', 'rot13' ),
'rot13_setting_callback_function',
'rot13-words-blacklist',
'rot13_setting_section'
);
// Register our setting so that $_POST handling is done for us
register_setting( 'rot13_settings_group', 'rot13_plugin_option', 'sanitize_text_field' );
The three functions above must be enclosed in a function and hooked to the admin_init
action, like so:
/**
* Hook the Settings API to 'admin_init' action
*/
function rot13_settings_api_init() {
// Add the section
add_settings_section(
'rot13_setting_section',
’,
'rot13_setting_section_callback_function',
'rot13-words-blacklist'
);
// Add the textarea field to the section
add_settings_field(
'blacklisted_words',
__( 'Blacklisted words', 'rot13' ),
'rot13_setting_callback_function',
'rot13-words-blacklist',
'rot13_setting_section'
);
// Register our setting so that $_POST handling is done for us
register_setting( 'rot13_settings_group', 'rot13_plugin_option', 'sanitize_text_field' );
}
add_action( 'admin_init', 'rot13_settings_api_init' );
Lest I forget, here is the code for the rot13_setting_callback_function()
and rot13_setting_section_callback_function()
functions, which will output the textarea
field and the description of the field (at the top of the section), respectively.
/**
* Add a description of the field to the top of the section
*/
function rot13_setting_section_callback_function() {
echo '<p>' . __( 'Enter a list of words to blacklist, separated by commas (,)', 'rot13' ) . '</p>';
}
/**
* Callback function to output the textarea form field
*/
function rot13_setting_callback_function() {
echo '<textarea rows="10" cols="60" name="rot13_plugin_option" class="code">' . esc_textarea( get_option( 'rot13_plugin_option' ) ) . '</textarea>';
}
At this point, we are done building the settings page for the plugin.
Up next is getting the plugin to detect blacklisted words and encrypt them with ROT13.
Detecting Blacklisted Words and Encrypting In ROT13
Here is an overview of how we will detect blacklisted words in a WordPress post:
- A post’s contents are broken down into individual words and saved to an array (
$post_words
). - The blacklisted words that were saved by the plugin to the database are retrieved. They, too, are broken down into individual words and saved to an array (
$blacklisted_words
). - We iterate over the
$post_words
arrays and check for any word that is on the blacklist. - If a blacklisted word is found, then
str_rot13()
encodes it in ROT13.
It’s time to create the PHP function (rot13_filter_post_content()
) that filters the contents of a post and then actually detects blacklisted words and encrypts them in ROT13.
Below is the code for the post’s filter.
/**
* Encrypt every blacklisted word in ROT13
*
* @param $content string post content to filter
*
* @return string
*/
function rot13_filter_post_content( $content ) {
// Get the words marked as blacklisted by the plugin
$blacklisted_words = esc_textarea( get_option( 'rot13_plugin_option' ) );
// If no blacklisted word are defined, return the post's content.
if ( empty( $blacklisted_words ) ) {
return $content;
}
else {
// Ensure we are dealing with "posts", not "pages" or any other content type.
if ( is_singular( 'post' ) ) {
// Confine each word in a post to an array
$post_words = preg_split( "/\b/", $content );
// Break down the post's contents into individual words
$blacklisted_words = explode( ',', $blacklisted_words );
// Remove any leading or trailing white space
$blacklisted_words = array_map(
function ( $arg ) {
return trim( $arg );
},
$blacklisted_words
);
// Iterate over the array of words in the post
foreach ( $post_words as $key => $value ) {
// Iterate over the array of blacklisted words
foreach ( $blacklisted_words as $words ) {
// Compare the words, being case-insensitive
if ( strcasecmp( $post_words[ $key ], $words ) == 0 ) {
// Encrypt any blacklisted word
$post_words[ $key ] = '<del>' . str_rot13( $value ) . '</del>';
}
}
}
// Convert the individual words in the post back into a string or text
$content = implode( ’, $post_words );
}
return $content;
}
}
add_filter( 'the_content', 'rot13_filter_post_content' );
While the code above for the filter
function is quite easy to understand, especially because it is so heavily commented, I’ll explain a bit more anyway.
The is_singular( ‘post’ )
conditional tag ensures that we are dealing with a post, and not a page or any other content type.
With preg_split()
, we are breaking down the post’s contents into individual words and saving them as an array by searching for the RegEx pattern \b
, which matches word boundaries.
The list of blacklisted words is retrieved from the database using get_option()
, with rot13_plugin_option
as the option’s name.
From the screenshot of the plugin’s settings page above and the description of the textarea
field, we can see that the blacklisted words are separated by commas, our delimiter. The explode
PHP function breaks down the blacklisted words into an array by searching for those commas.
A closure is applied to the $blacklisted_words
array via array_map()
that will trim leading and trailing white spaces from the array values (the individual blacklisted words).
The foreach
construct iterates over the post’s words and check whether any word is in the array of blacklisted words. Any blacklisted word that gets detected is encrypted in ROT13 and enclosed in a <del>
tag.
The $post_words
array is converted back to a string or text and subsequently returned.
Finally, the function is hooked to the the_content
filter action.
Below is a screenshot of a post with the words “love” and “forever” blacklisted.
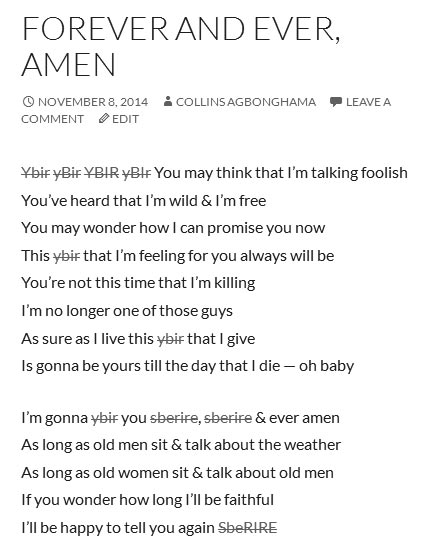
Wrapping Up
ROT13 is a simple encryption technique that can be easily decrypted. Thus, you should never use it for serious data encryption.
Even if you don’t end up using the plugin, the concepts you’ve learned in creating it can be applied to many situations, such as obfuscating or encrypting inappropriate words (such as profanities) in ROT13, which would be a nice feature in a forum where people have the freedom to post anything.
Hopefully, you have learned a thing or two from this tutorial. If you have any question or a contribution, please let us know in the comments.
Further Reading
- A Look At The Modern WordPress Server Stack
- Secrets Of High-Traffic WordPress Blogs
- Speeding Up Your Website’s Database
- Do-It-Yourself Caching Methods With WordPress
Front page image credit: Wikipedia.
