How To Use Autoloading And A Plugin Container In WordPress Plugins
Building and maintaining a WordPress plugin can be a daunting task. The bigger the codebase, the harder it is to keep track of all the working parts and their relationship to one another. And you can add to that the limitations imposed by working in an antiquated version of PHP, 5.2.
In this article we will explore an alternative way of developing WordPress plugins, using the lessons learned from the greater PHP community, the world outside WordPress. We will walk through the steps of creating a plugin and investigate the use of autoloading and a plugin container.
Let’s Begin
The first thing you need to do when creating a plugin is to give it a unique name. The name is important as it will be the basis for all our unique identifiers (function prefix, class prefix, textdomain, option prefix, etc.). The name should also be unique across the wordpress.org space. It won’t hurt if we make the name catchy. For our sample plugin I chose the name Simplarity, a play on the words “simple” and “clarity”.
We’ll assume you have a working WordPress installation already.
Folder Structure
First, create a directory named simplarity inside wp-content/plugins. Inside it create the following structure:
- simplarity.php: our main plugin file
- css/: directory containing our styles
- js/: directory containing JavaScript files
- languages/: directory that will contain translation files
- src/: directory containing our classes
- views/: directory that will contain our plugin view files
The Main Plugin File
Open the main plugin file, simplarity.php, and add the plugin information header:
<?php
/*
Plugin Name: Simplarity
description: >-
A plugin for smashingmagazine.com
Version: 1.0.0
License: GPL-2.0+
*/
This information is enough for now. The plugin name, description, and version will show up in the plugins area of WordPress admin. The license details are important to let your users know that this is an open source plugin. A full list of header information can found in the WordPress codex.
Autoloading
Autoloading allows you to automatically load classes using an autoloader so you don’t have to manually include the files containing the class definitions. For example, whenever you need to use a class, you need to do the following:
require_once '/path/to/classes/class-container.php';
require_once '/path/to/classes/class-view.php';
require_once '/path/to/classes/class-settings-page.php';
$plugin = new Container();
$view = new View();
$settings_page = new SettingsPage();
With autoloading, you can use an autoloader instead of multiple require_once
statements. It also eliminates the need to update these require statements whenever you add, rename, or change the location of your classes. That’s a big plus for maintainability.
Adopting The PEAR Naming Convention For Class Names
Before we create our autoloader we need to create a convention for our class names and their location in the file system. This will aid the autoloader in mapping out the class to its source file.
For our class names we will adopt the PEAR naming convention. The gist is that class names are alphabetic characters in StudlyCaps. Each level of the hierarchy is separated with a single underscore. Class names will directly map to the directories in which they are stored.
It’s easier to illustrate it using examples:
- A class named Simplarity_Plugin would be defined in the file src/Simplarity/Plugin.php.
- A class named Simplarity_SettingsPage would be defined in src/Simplarity/SettingsPage.php.
As you can see with this convention, the autoloader will just replace the underscores with directory separators to locate the class definition.
What About The WordPress Coding Standards For Class Names?
As you might be aware, WordPress has its own naming convention for class names. It states:
"Class names should use capitalized words separated by underscores. Any acronyms should be all upper case. […] Class file names should be based on the class name withclass-
prepended and the underscores in the class name replaced with hyphens, for exampleWP_Error
becomesclass-wp-error.php
"
I know that we should follow the standards of the platform that we are developing on. However, we suggest using the PEAR naming convention because:
- WP coding standards do not cover autoloading.
- WP does not follow its own coding standards. Examples: class.wp-scripts.php and SimplePie. This is understandable since WordPress grew organically.
- Interoperability allows you to easily use third-party libraries that follow the PEAR naming convention, like Twig. And conversely, you can easily port your code to other libraries sharing the same convention.
- It’s important your autoloader is future-ready. When WordPress decides to up the ante and finally move to PHP 5.3 as its minimum requirement, you can easily update the code to be PSR-0 or PSR-4-compatible and take advantage of the built-in namespaces instead of using prefixes. This is a big plus for interoperability.
Note that we are only using this naming convention for classes. The rest of our code will still follow the WordPress coding standards. It’s important to follow and respect the standards of the platform that we are developing on.
Now that we have fully covered the naming convention, we can finally build our autoloader.
Building Our Autoloader
Open our main plugin file and add the following code below the plugin information header:
spl_autoload_register( 'simplarity_autoloader' );
function simplarity_autoloader( $class_name ) {
if ( false !== strpos( $class_name, 'Simplarity' ) ) {
$classes_dir = realpath( plugin_dir_path( __FILE__ ) ) . DIRECTORY_SEPARATOR . 'src' . DIRECTORY_SEPARATOR;
$class_file = str_replace( '_', DIRECTORY_SEPARATOR, $class_name ) . '.php';
require_once $classes_dir . $class_file;
}
}
At the heart of our autoloading mechanism is PHP’s built in spl_autoload_register
function. All it does is register a function to be called automatically when your code references a class that hasn’t been loaded yet.
The first line tells spl_autoload_register
to register our function named simplarity_autoloader
:
spl_autoload_register( 'simplarity_autoloader' );
Next we define the simplarity_autoloader function:
function simplarity_autoloader( $class_name ) {
…
}
Notice that it accepts a $class_name
parameter. This parameter holds the class name. For example when you instantiate a class using $plugin = new Simplarity_Plugin()
, $class_name
will contain the string “SimplarityPlugin”. Since we are adding this function in the global space, it’s important that we have it prefixed with simplarity
.
The next line checks if $classname
contains the string “Simplarity” which is our top level namespace:
if ( false !== strpos( $class_name, 'Simplarity' ) ) {
This will ensure that the autoloader will only run on our classes. Without this check, our autoloader will run every time an unloaded class is referenced, even if the class is not ours, which is not ideal.
The next line constructs the path to the directory where our classes reside:
$classes_dir = realpath( plugin_dir_path( __FILE__ ) ) . DIRECTORY_SEPARATOR . 'src' . DIRECTORY_SEPARATOR;
It uses WP’s plugin_dir_path
to get the plugin root directory. FILE
is a magic constant that contains the full path and filename of the current file. DIRECTORY_SEPARATOR
is a predefined constant that contains either a forward slash or backslash depending on the OS your web server is on. We also use realpath
to normalize the file path.
This line resolves the path to the class definition file:
$class_file = str_replace( '_', DIRECTORY_SEPARATOR, $class_name ) . '.php';
It replaces the underscore (_
) in $class_name
with the directory separator and appends .php
.
Finally, this line builds the file path to the definition and includes the file using require_once
:
require_once $classes_dir . $class_file;
That’s it! You now have an autoloader. Say goodbye to long lines of require_once
statements.
Plugin Container
A plugin container is a special class that holds together our plugin code. It simplifies the interaction between the many working parts of your code by providing a centralized location to manage the configuration and objects.
Uses Of Our Plugin Container
Here are the things we can expect from the plugin container:
- Store global parameters in a single location
Often you’ll find this code in plugins:
define( 'SIMPLARITY_VERSION', '1.0.0' );
define( 'SIMPLARITY_PATH', realpath( plugin_dir_path( __FILE__ ) ) . DIRECTORY_SEPARATOR );
define( 'SIMPLARITY_URL', plugin_dir_url( __FILE__ ) );
Instead of doing that, we could do this instead:
$plugin = new Simplarity_Plugin();
$plugin['version] = '1.0.0';
$plugin['path'] = realpath( plugin_dir_path( __FILE__ ) ) . DIRECTORY_SEPARATOR;
$plugin['url'] = plugin_dir_url( __FILE__ );
This has the added benefit of not polluting the global namespace with our plugin’s constants, which in most cases aren’t needed by other plugins.
- Store objects in a single location
Instead of scattering our class instantiations everywhere in our codebase we can just do this in a single location:
$plugin = new Simplarity_Plugin();
/…/
$plugin['scripts'] = new Simplarity_Scripts(); // A class that loads javascript files
- Service definitions
This is the most powerful feature of the container. A service is an object that does something as part of a larger system. Services are defined by functions that return an instance of an object. Almost any global object can be a service.
$plugin['settings_page'] = function ( $plugin ) {
return new SettingsPage( $plugin['settings_page_properties'] );
};
Services result in lazy initialization whereby objects are only instantiated and initialized when needed. It also allows us to easily implement a self-resolving dependency injection design. An example:
$plugin = new Plugin();
$plugin['door_width'] = 100;
$plugin['door_height'] = 500;
$plugin['door_size'] = function ( $plugin ) {
return new DoorSize( $plugin['door_width'], $plugin['door_height'] );
};
$plugin['door'] = function ( $plugin ) {
return new Door( $plugin['door_size'] );
};
$plugin['window'] = function ( $plugin ) {
return new Window();
};
$plugin['house'] = function ( $plugin ) {
return new House( $plugin['door'], $plugin['window'] );
};
$house = $plugin['house'];
This is roughly equivalent to:
$door_width = 100;
$door_height = 500;
$door_size = new DoorSize( $door_width, $door_height );
$door = new Door( $door_size );
$window = new Window();
$house = new House( $door, $window );
Whenever we get an object, as in `$house = $plugin['house'];` , the object is created (lazy initialization) and dependencies are resolved automatically.</p>
Building The Plugin Container
Let’s start by creating the plugin container class. We will name it “Simplarity_Plugin”. As our naming convention dictates, we should create a corresponding file: src/Simplarity/Plugin.php.
Open Plugin.php and add the following code:
<?php
class Simplarity_Plugin implements ArrayAccess {
protected $contents;
public function __construct() {
$this->contents = array();
}
public function offsetSet( $offset, $value ) {
$this->contents[$offset] = $value;
}
public function offsetExists($offset) {
return isset( $this->contents[$offset] );
}
public function offsetUnset($offset) {
unset( $this->contents[$offset] );
}
public function offsetGet($offset) {
if( is_callable($this->contents[$offset]) ){
return call_user_func( $this->contents[$offset], $this );
}
return isset( $this->contents[$offset] ) ? $this->contents[$offset] : null;
}
public function run(){
foreach( $this->contents as $key => $content ){ // Loop on contents
if( is_callable($content) ){
$content = $this[$key];
}
if( is_object( $content ) ){
$reflection = new ReflectionClass( $content );
if( $reflection->hasMethod( 'run' ) ){
$content->run(); // Call run method on object
}
}
}
}
}
The class implements the ArrayAccess
interface:
class Simplarity_Plugin implements ArrayAccess {
This allows us to use it like PHP’s array:
$plugin = new Simplarity_Plugin();
$plugin['version'] = '1.0.0'; // Simplicity is beauty
The functions offsetSet
, offsetExists
, offsetUnset
and offsetGet
are required by ArrayAccess
to be implemented. The run
function will loop through the contents of the container and run the runnable objects.
To better illustrate our plugin container, let’s start by building a sample plugin.
Example Plugin: A Settings Page
This plugin will add a settings page named “Simplarity” under WordPress Admin → Settings.
Let’s go back to the main plugin file. Open up simplarity.php and add the following code. Add this below the autoloader code:
add_action( 'plugins_loaded', 'simplarity_init' ); // Hook initialization function
function simplarity_init() {
$plugin = new Simplarity_Plugin(); // Create container
$plugin['path'] = realpath( plugin_dir_path( __FILE__ ) ) . DIRECTORY_SEPARATOR;
$plugin['url'] = plugin_dir_url( __FILE__ );
$plugin['version'] = '1.0.0';
$plugin['settings_page_properties'] = array(
'parent_slug' => 'options-general.php',
'page_title' => 'Simplarity',
'menu_title' => 'Simplarity',
'capability' => 'manage_options',
'menu_slug' => 'simplarity-settings',
'option_group' => 'simplarity_option_group',
'option_name' => 'simplarity_option_name'
);
$plugin['settings_page'] = new Simplarity_SettingsPage( $plugin['settings_page_properties'] );
$plugin->run();
}
Here we use WP’s add_action
to hook our function simplarity_init
into plugins_loaded
:
add_action( 'plugins_loaded', 'simplarity_init' );
This is important as this will make our plugin overridable by using remove_action
. An example use case would be a premium plugin overriding the free version.
Function simplarity_init
contains our plugin’s initialization code. At the start, we simply instantiate our plugin container:
$plugin = new Simplarity_Plugin();
These lines assign global configuration data:
$plugin['path'] = realpath( plugin_dir_path( __FILE__ ) ) . DIRECTORY_SEPARATOR;
$plugin['url'] = plugin_dir_url( __FILE__ );
$plugin['version'] = '1.0.0';
The plugin path contains the full path to our plugin, the url
contains the URL to our plugin directory. They will come in handy whenever we need to include files and assets. version
contains the current version of the plugin that should match the one in the header info. Useful whenever you need to use the version in code.
This next code assigns various configuration data to settings_page_properties
:
$plugin['settings_page_properties'] = array(
'parent_slug' => 'options-general.php',
'page_title' => 'Simplarity',
'menu_title' => 'Simplarity',
'capability' => 'manage_options',
'menu_slug' => 'simplarity-settings',
'option_group' => 'simplarity_option_group',
'option_name' => 'simplarity_option_name'
);
These configuration data are related to WP settings API.
This next code instantiates the settings page, passing along settings_page_properties
:
$plugin['settings_page'] = new Simplarity_SettingsPage( $plugin['settings_page_properties'] );
The run
method is where the fun starts:
$plugin->run();
It will call Simplarity_SettingsPage
’s own run
method.
The Simplarity_SettingsPage
Class
Now we need to create the Simplarity_SettingsPage
class. It’s a class that groups together the settings API functions.
Create a file named SettingsPage.php in src/Simplarity/. Open it and add the following code:
<?php
class Simplarity_SettingsPage {
protected $settings_page_properties;
public function __construct( $settings_page_properties ){
$this->settings_page_properties = $settings_page_properties;
}
public function run() {
add_action( 'admin_menu', array( $this, 'add_menu_and_page' ) );
add_action( 'admin_init', array( $this, 'register_settings' ) );
}
public function add_menu_and_page() {
add_submenu_page(
$this->settings_page_properties['parent_slug'],
$this->settings_page_properties['page_title'],
$this->settings_page_properties['menu_title'],
$this->settings_page_properties['capability'],
$this->settings_page_properties['menu_slug'],
array( $this, 'render_settings_page' )
);
}
public function register_settings() {
register_setting(
$this->settings_page_properties['option_group'],
$this->settings_page_properties['option_name']
);
}
public function get_settings_data(){
return get_option( $this->settings_page_properties['option_name'], $this->get_default_settings_data() );
}
public function render_settings_page() {
$option_name = $this->settings_page_properties['option_name'];
$option_group = $this->settings_page_properties['option_group'];
$settings_data = $this->get_settings_data();
?>
<div class="wrap">
<h2>Simplarity</h2>
<p>This plugin is using the settings API.</p>
<form method="post" action="options.php">
<?php
settings_fields( $this->plugin['settings_page_properties']['option_group']);
?>
<table class="form-table">
<tr>
<th><label for="textbox">Textbox:</label></th>
<td>
<input type="text" id="textbox"
name="<?php echo esc_attr( $option_name."[textbox]" ); ?>"
value="<?php echo esc_attr( $settings_data['textbox'] ); ?>" />
</td>
</tr>
</table>
<input type="submit" name="submit" id="submit" class="button button-primary" value="Save Options">
</form>
</div>
<?php
}
public function get_default_settings_data() {
$defaults = array();
$defaults['textbox'] = ’;
return $defaults;
}
}
The class property $settings_page_properties
stores the settings related to WP settings API:
<?php
class Simplarity_SettingsPage {
protected $settings_page_properties;
The constructor function accepts the settings_page_properties
and stores it:
public function __construct( $settings_page_properties ){
$this->settings_page_properties = $settings_page_properties;
}
The values are passed from this line in the main plugin file:
$plugin['settings_page'] = new Simplarity_SettingsPage( $plugin['settings_page_properties'] );
The run
function is use to run startup code:
public function run() {
add_action( 'admin_menu', array( $this, 'add_menu_and_page' ) );
add_action( 'admin_init', array( $this, 'register_settings' ) );
}
The most likely candidate for startup code are filters and action hooks. Here we add the action hooks related to our settings page. Do not confuse this run method with the run method of the plugin container. This run method belongs to the settings page class.
This line hooks the add_menu_and_page
function on to the admin_menu
action:
add_action( 'admin_menu', array( $this, 'add_menu_and_page' ) );
Function add_submenu_page
in turn calls WP’s add_submenu_page
function to add a link under the WP Admin → Settings:
public function add_menu_and_page() {
add_submenu_page(
$this->settings_page_properties['parent_slug'],
$this->settings_page_properties['page_title'],
$this->settings_page_properties['menu_title'],
$this->settings_page_properties['capability'],
$this->settings_page_properties['menu_slug'],
array( $this, 'render_settings_page' )
);
}
As you can see, we are pulling the info from our class property $settings_page_properties
which we specified in the main plugin file.
The parameters for add_submenu_page
are:
parent_slug
: slug name for the parent menupage_title
: text to be displayed in the<title>
element of the page when the menu is selectedmenu_title
: text to be used for the menucapability
: the capability required for this menu to be displayed to the usermenu_slug
: slug name to refer to this menu by (should be unique for this menu)function
: function to be called to output the content for this page
This line hooks the register_settings
function on to the admin_init
action:
add_action( 'admin_init', array( $this, 'register_settings' ) );
array( $this, ‘register_settings’ )
means to call register_settings
on $this
, which points to our SettingsPage
instance.
The register_settings
then calls WP’s register_setting
to register a setting:
public function register_settings() {
register_setting(
$this->settings_page_properties['option_group'],
$this->settings_page_properties['option_name']
);
}
Function render_settings_page
is responsible for rendering the page:
public function render_settings_page() {
$option_name = $this->settings_page_properties['option_name'];
$option_group = $this->settings_page_properties['option_group'];
$settings_data = $this->get_settings_data();
?>
<div class="wrap">
<h2>Simplarity</h2>
<p>This plugin is using the settings API.</p>
<form method="post" action="options.php">
<?php
settings_fields( $option_group );
?>
<table class="form-table">
<tr>
<th><label for="textbox">Textbox:</label></th>
<td>
<input type="text" id="textbox"
name="<?php echo esc_attr( $option_name."[textbox]" ); ?>"
value="<?php echo esc_attr( $settings_data['textbox'] ); ?>" />
</td>
</tr>
</table>
<input type="submit" name="submit" id="submit" class="button button-primary" value="Save Options">
</form>
</div>
<?php
}
We hooked render_settings_page
earlier using add_submenu_page
.
Function get_settings_data
is a wrapper function for get_option
:
public function get_settings_data(){
return get_option( $this->plugin['settings_page_properties']['option_name'] );
}
This is to easily get the settings data with a single function call.
Function get_default_settings_data
is use to supply us with our own default values:
public function get_default_settings_data() {
$defaults = array();
$defaults['textbox'] = ’;
return $defaults;
}
Abstracting Our Settings Page Class
Right now our settings page class cannot be reused if you want to create another subpage. Let’s move the reusable code for the settings page to another class.
Let’s call this class Simplarity_WpSubPage
. Go ahead and create the file src/Simplarity/WpSubPage.php.
Now add the code below:
<?php
abstract class Simplarity_WpSubPage {
protected $settings_page_properties;
public function __construct( $settings_page_properties ){
$this->settings_page_properties = $settings_page_properties;
}
public function run() {
add_action( 'admin_menu', array( $this, 'add_menu_and_page' ) );
add_action( 'admin_init', array( $this, 'register_settings' ) );
}
public function add_menu_and_page() {
add_submenu_page(
$this->settings_page_properties['parent_slug'],
$this->settings_page_properties['page_title'],
$this->settings_page_properties['menu_title'],
$this->settings_page_properties['capability'],
$this->settings_page_properties['menu_slug'],
array( $this, 'render_settings_page' )
);
}
public function register_settings() {
register_setting(
$this->settings_page_properties['option_group'],
$this->settings_page_properties['option_name']
);
}
public function get_settings_data(){
return get_option( $this->settings_page_properties['option_name'], $this->get_default_settings_data() );
}
public function render_settings_page(){
}
public function get_default_settings_data() {
$defaults = array();
return $defaults;
}
}
Notice that it is an abstract class. This will prevent intantiating this class directly. To use it you need to extend it first with another class, which in our case is Simplarity_SettingsPage
:
<?php
class Simplarity_SettingsPage extends Simplarity_WpSubPage {
public function render_settings_page() {
$option_name = $this->settings_page_properties['option_name'];
$option_group = $this->settings_page_properties['option_group'];
$settings_data = $this->get_settings_data();
?>
<div class="wrap">
<h2>Simplarity</h2>
<p>This plugin is using the settings API.</p>
<form method="post" action="options.php">
<?php
settings_fields( $option_group );
?>
<table class="form-table">
<tr>
<th><label for="textbox">Textbox:</label></th>
<td>
<input type="text" id="textbox"
name="<?php echo esc_attr( $option_name."[textbox]" ); ?>"
value="<?php echo esc_attr( $settings_data['textbox'] ); ?>" />
</td>
</tr>
</table>
<input type="submit" name="submit" id="submit" class="button button-primary" value="Save Options">
</form>
</div>
<?php
}
public function get_default_settings_data() {
$defaults = array();
defaults['textbox'] = ’;
return $defaults;
}
}
The only functions we have implemented are render_settings_page
and get_default_settings_data
, which are customized to this settings page.
To create another WP settings page you’ll just need to create a class and extend the Simplarity_WpSubPage
. And implement your own render_settings_page
and get_default_settings_data
.
Defining A Service
The power of the plugin container is in defining services. A service is a function that contains instantiation and initialization code that will return an object. Whenever we pull a service from our container, the service function is called and will create the object for you. The object is only created when needed. This is called lazy initialization.
To better illustrate this, let’s define a service for our settings page.
Open simplarity.php and add this function below the Simplarity code:
function simplarity_service_settings( $plugin ){
$object = new Simplarity_SettingsPage( $plugin['settings_page_properties'] );
return $object;
}
Notice that our service function has a $plugin
parameter which contains our plugin container. This allows us to access all configuration, objects, and services that have been stored in our plugin container. We can see that the Simplarity_SettingsPage
has a dependency on $plugin[‘settings_page_properties’]
. We inject this dependency to Simplarity_SettingsPage
here. This is an example of dependency injection. Dependency injection is a practice where objects are designed in a manner where they receive instances of the objects from other pieces of code, instead of constructing them internally. This improves decoupling of code.
Now let’s replace this line in simplarity_init
:
$plugin['settings_page'] = new Simplarity_SettingsPage( $plugin['settings_page_properties'] );
with a service definition assignment:
$plugin['settings_page'] = 'simplarity_service_settings'
So instead of assigning our object instance directly, we assign the name of our function as string. Our container handles the rest.
Defining A Shared Service
Right now, every time we get $plugin[‘settings_page’]
, a new instance of Simplarity_SettingsPage
is returned. Ideally, Simplarity_SettingsPage
should only be instantiated once as we are using WP hooks, which in turn should only be registered once.
To solve this we use a shared service. A shared service will return a new instance of an object on first call, on succeeding calls it will return the same instance.
Let’s create a shared service using a static variable:
function simplarity_service_settings( $plugin ){
static $object;
if (null !== $object) {
return $object;
}
$object = new Simplarity_SettingsPage( $plugin['settings_page_properties'] );
return $object;
}
On first call, $object
is null, and on succeeding calls it will contain the instance of the object created on first call. Notice that we are using a static variable. A static variable exists only in a local function scope, but it does not lose its value when program execution leaves this scope.
That’s it.
Now if you activate the plugin, an admin menu will appear in Admin → Settings named “Simplarity”. Click on it and you will be taken to the settings page we have created.
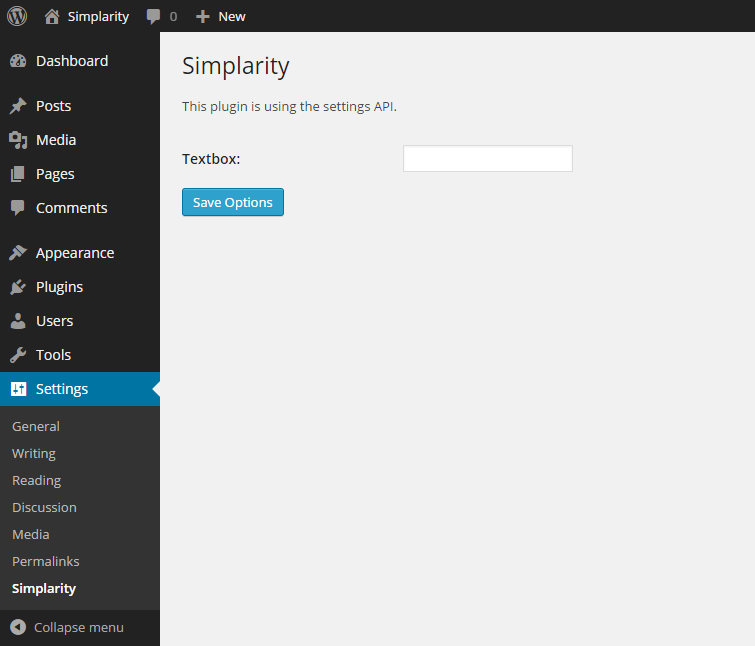
The Future: PHP 5.3+
Earlier we mentioned that our class naming convention was future-ready. In this section we will discuss how our codebase will work in PHP version 5.3 and up. Two of the best features that have graced the PHP world are namespaces and anonymous functions.
Namespaces
PHP does not allow two classes or functions to share the same name. When this happens, a name collision occurs and causes a nasty error.
With namespaces you can have the same class names as long as they live in their own namespace. A good analogy for namespaces are the folders you have in your OS. You cannot have files with the same name in one folder. However, you can have the same filenames in different folders.
With namespaces, class and function names won’t need unique prefixes anymore.
Anonymous Functions
Anonymous functions, also known as closures, allow the creation of functions which have no specified name. They are most useful as the value of callback parameters, but they have many other uses. You can also store closures in variables.
Here’s an example of closure:
<?php
$greet = function($name) {
printf("Hello %s\r\n", $name);
};
$greet('World');
$greet('PHP');
Using Namespaces In Classes
Let’s go ahead and use namespaces in our class definitions. Open up the following files in src/Simplarity:
- Plugin.php
- SettingsPage.php
- WpSubPage.php
In each of these files, add a namespace declaration on top and remove the “Simplarity_” prefix on class names:
// Plugin.php
namespace Simplarity;
class Plugin {
...
// SettingsPage.php
namespace Simplarity;
class SettingsPage extends WpSubPage {
...
// WpSubPage.php
namespace Simplarity;
abstract class WpSubPage {
...
Since we have updated our class names we also need to update our class instantiations in simplarity.php. We do this by deleting the prefixes:
function simplarity_init() {
$plugin = new Plugin();
...
}
...
function simplarity_service_settings( $plugin ){
...
$object = new SettingsPage( $plugin['settings_page_properties'] );
return $object;
}
By default, PHP will try to load the class from the root namespace so we need to tell it about our namespaced classes. We add this to the top of simplarity.php just above the autoloader code:
use Simplarity\Plugin;
use Simplarity\SettingsPage;
This is called importing/aliasing with the use operator.
Updating The Autoloader
Open up simplarity.php and change this line in the autoloader from:
$class_file = str_replace( '_', DIRECTORY_SEPARATOR, $class_name ) . '.php';
to:
$class_file = str_replace( '\\', DIRECTORY_SEPARATOR, $class_name ) . '.php';
Remember that in 5.2 code we are using underscores as hierarchy separators. For 5.3+ we are using namespaces which use backslash “” as hierarchy separators. Thus we simply swap “_” for “”. We use another backslash to escape the original one: “”.
Updating Our Service Definitions To Use Anonymous Functions
We can now replace the global functions we created for our service definitions with anonymous functions. So instead of doing this:
function simplarity_init() {
...
$plugin['settings_page'] = 'simplarity_service_settings';
...
}
...
function simplarity_service_settings( $plugin ){
static $object;
if (null !== $object) {
return $object;
}
$object = new Simplarity_SettingsPage( $plugin['settings_page_properties'] );
return $object;
}
we can just replace this with an inline anonymous function:
function simplarity_init() {
$plugin = new Plugin();
...
$plugin['settings_page'] = function ( $plugin ) {
static $object;
if (null !== $object) {
return $object;
}
return new SettingsPage( $plugin['settings_page_properties'] );
};
...
}
Using Pimple As A Plugin Container
Pimple is a small dependency injection (DI) container for PHP 5.3+. Pimple has the same syntax as our simple plugin container. In fact our plugin container was inspired by Pimple. In this part, we will extend Pimple and use it.
Download Pimple container from GitHub and save it in src/Simplarity/Pimple.php.
Open up Pimple.php and replace the namespace and the classname to:
...
namespace Simplarity;
/**
* Container main class.
*
* @author Fabien Potencier
*/
class Pimple implements \ArrayAccess
...
Open up Plugin.php and replace all the code with:
<?php
namespace Simplarity;
class Plugin extends Pimple {
public function run(){
foreach( $this->values as $key => $content ){ // Loop on contents
$content = $this[$key];
if( is_object( $content ) ){
$reflection = new \ReflectionClass( $content );
if( $reflection->hasMethod( 'run' ) ){
$content->run(); // Call run method on object
}
}
}
}
}
Now let’s change the service definition in simplarity.php to:
$plugin['settings_page'] = function ( $plugin ) {
return new SettingsPage( $plugin['settings_page_properties'] );
};
By default, each time you get a service, Pimple returns the same instance of it. If you want a different instance to be returned for all calls, wrap your anonymous function with the factory()
method:
$plugin['image_resizer'] = $plugin->factory(function ( $plugin ) {
return new ImageResizer( $plugin['image_dir'] );
});
Conclusion
The PHP community is big. A lot of best practices have been learned over the years. It’s good to always look beyond the walled garden of WordPress to look for answers. With autoloading and a plugin container we are one step closer to better code.
Code Samples
Resources
Further Reading
- Ten Things Every WordPress Plugin Developer Should Know
- Making A WordPress Plugin That Uses Service APIs
- How Commercial Plugin Developers Are Using The Repository
- How To Improve Your WordPress Plugin’s Readme.txt
