Creating Universal Windows Apps With React Native
React.js is a popular JavaScript library for building reusable UI components. React Native takes all the great features of React, from the one-way binding and virtual DOM to debugging tools, and applies them to mobile app development on iOS and Android.
With the React Native Universal Windows platform extension, you can now make your React Native applications run on the Universal Windows families of devices, including desktop, mobile, and Xbox, as well as Windows IoT, Surface Hub, and HoloLens.
In this code story, we will walk through the process of setting up a Universal Windows project for React Native, importing core Windows-specific modules to your JavaScript components, and running the app with Visual Studio.
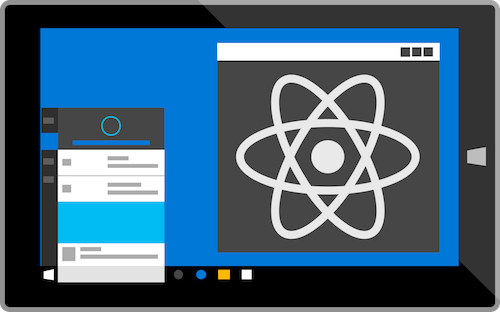
Installing React Native For Windows
Installing the React Native Universal Windows platform extension is easy, whether you want to add the Windows platform to your existing app, or you want to start from scratch building an app just for Windows.
Adding React Native For Windows To Existing Projects
React Native developers may be familiar with RNPM, a tool initially built to simplify the process of adding native dependencies to React Native projects. RNPM has a great plugin architecture upon which the React Native for Windows command line tools were built.
To start, make sure you have RNPM installed globally.
npm install -g rnpm
Once RNPM is installed, install the Windows plugin for RNPM and initialize your project.
npm install --save-dev rnpm-plugin-windows
rnpm windows
The windows
command will do the following:
- Install
react-native-windows
from NPM, - Read the name of your project from
package.json
, - Use Yeoman to generate the Windows project files.
The RNPM plugin architecture searches your local package.json dependencies
and devDependencies
for modules that match rnpm-plugin-*
, hence the –save-dev
above.
Head to GitHub for more information on rnpm-plugin-windows
.
Creating A React Native For Windows Project From Scratch
You have a few different options to create a React Native Universal Windows project from scratch. If your eventual intent is to also build apps for iOS and Android in the same code base, then the recommendation is to first use the existing tutorial to set up your React Native project, and then follow the steps above. E.g.,
<code="language-bash">npm install -g react-native-cli react-native init myapp
Note: the react-native-windows
NPM package is only compatible with the latest versions of react-native
, from version 0.27 onwards.
Otherwise, you can easily set yourself up with npm init
.
mkdir myapp
cd myapp
npm init
npm install --save-dev rnpm-plugin-windows
rnpm windows
Note: the default behavior for choosing the version of react-native-windows
is to install the latest version if the package.json
does not yet have a react-native
dependency. Otherwise, if a react-native
dependency already exists, the plugin will attempt to install a version of react-native-windows
that matches the major and minor version of react-native
.
The Windows plugin for RNPM will automatically install the react-native
and react
peer dependencies for react-native-windows
if they have not yet been installed.
React Native For Windows Project Structure
There are a few boilerplate files that get generated for the Universal Windows App. The important ones include:
index.windows.js
— This is the entry point to your React application.windows/myapp.sln
— This is where you should start to launch your app and debug native code.windows/myapp/MainPage.cs
— This is where you can tweak the native bridge settings, like available modules and components.
Here’s the full output:
<code="language-bash">├── index.windows.js ├── windows ├── myapp.sln ├── myapp ├── Properties ├── AssemblyInfo.cs ├── Default.rd.xml ├── Assets ├── ... ├── App.xaml ├── App.xaml.cs ├── MainPage.cs ├── project.json ├── Package.appxmanifest ├── myapp_TemporaryKey.pfx
We ship the core C# library for React Native as source on NPM. Having direct access to the source will help with debugging and making small tweaks where necessary. As long as the core ships as source, third party modules will also need to follow suit (although their dependencies may be binary). We’ll be working with the React Native community on react-native link
support to make dependency management as simple as possible. Instructions for how to link dependencies are available on GitHub.
Building And Extending Apps For The Windows Platform
The core components and modules for React Native are imported as follows:
import {
View,
Text,
Image
} from 'react-native';
Currently, the same goes for Android- and iOS-specific modules, i.e.:import {
TabBarIOS,
DrawerLayoutAndroid
} from 'react-native';
Since Windows is a plugin to the framework, core modules specific to Windows are imported from react-native-windows
, i.e.:import {
FlipViewWindows,
SplitViewWindows
} from 'react-native-windows';
So, a typical imports section of a React component with a mixture of core and Windows-specific modules will look like:import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
View
} from 'react-native';
import {
SplitViewWindows
} from 'react-native-windows';
There is a list of the core modules available on React Native for Windows on GitHub.
There are multiple ways to add conditional behavior to your apps based on the platform. One way that you might already recognize is the use of the *.platform.js
pattern, i.e., index.[android|ios|windows].js
. The node-haste
dependency graph will choose the filename that matches the active platform choice, and fallback to the filename without any platform indicator, if available. E.g., if you have files MyComponent.windows.js
and MyComponent.js
, node-haste
will choose MyComponent.js
for Android and iOS and MyComponent.windows.js
for Windows.
Another way is to use conditional logic inside a component, as demonstrated in the simple component below.import React, {Component} from 'react';
import {
Platform,
Text,
View
} from 'react-native';
class HelloComponent extends Component { render() { var text = "Hello world!"; if (Platform.OS === 'android') { text = "Hello Android!"; } else if (Platform.OS === 'windows') { text = "Hello Windows!"; }
```
return (
<View>
<Text>{text}</text>
```
</View> ); } }
Facebook has a very good article on cross-platform design with React Native at makeitopen.com.
## Running The Universal Windows App
There are a few options for running your React Native Universal Windows app. The easiest way, for now, is to launch the app in Visual Studio. After initializing the project, open the Visual Studio solution at ./windows/myapp.sln
in Visual Studio 2015 (Community edition is supported). From Visual Studio, choose which platform you want to build for (x86, x64, or ARM), choose the target you want to deploy to, and press F5. We are still working on deployment from the command line, but work to enable a run-windows
command to the RNPM plugin is underway.
The instructions for running React Native UWP apps, both from Visual Studio and from the CLI, will be evolving on GitHub. If you have any problems getting started with building or running your React Windows application, reach out on Discord or open an issue. We look forward to hearing your feedback and reviewing your contributions!
## More Hands-On With Web And Mobile Apps
Check out these helpful resources on building web and Mobile Apps:Further Reading
- Beyond The Browser: From Web Apps To Desktop Apps
- Building Hybrid Apps With ChakraCore
- Providing A Native Experience With Web Technologies
- Server-Side Rendering With React, Node And Express
