Introducing Alpine.js: A Tiny JavaScript Framework
Like most developers, I have a bad tendency to over-complicate my workflow, especially if there’s some new hotness on the horizon. Why use CSS when you can use CSS-in-JS? Why use Grunt when you can use Gulp? Why use Gulp when you can use Webpack? Why use a traditional CMS when you can go headless? Every so often though, the new-hotness makes life simpler.
Recently, the rise of utility based tools like Tailwind CSS have done this for CSS, and now Alpine.js promises something similar for JavaScript.
In this article, we’re going to take a closer look at Alpine.js and how it can replace JQuery or larger JavaScript libraries to build interactive websites. If you regularly build sites that require a sprinkling on Javascript to alter the UI based on some user interaction, then this article is for you.
Throughout the article, I refer to Vue.js, but don’t worry if you have no experience of Vue — that is not required. In fact, part of what makes Alpine.js great is that you barely need to know any JavaScript at all.
Now, let’s get started.
What Is Alpine.js?
According to project author Caleb Porzio:
“Alpine.js offers you the reactive and declarative nature of big frameworks like Vue or React at a much lower cost. You get to keep your DOM, and sprinkle in behavior as you see fit.”
Let’s unpack that a bit.
Let’s consider a basic UI pattern like Tabs. Our ultimate goal is that when a user clicks on a tab, the tab contents displays. If we come from a PHP background, we could easily achieve this server side. But the page refresh on every tab click isn’t very ‘reactive’.
To create a better experience over the years, developers have reached for jQuery and/or Bootstrap. In that situation, we create an event listener on the tab, and when a user clicks, the event fires and we tell the browser what to do.
See the Pen Showing / hiding with jQuery by Phil on CodePen.
That works. But this style of coding where we tell the browser exactly what to do (imperative coding) quickly gets us in a mess. Imagine if we wanted to disable the button after it has been clicked, or wanted to change the background color of the page. We’d quickly get into some serious spaghetti code.
Developers have solved this issue by reaching for frameworks like Vue, Angular and React. These frameworks allow us to write cleaner code by utilizing the virtual DOM: a kind of mirror of the UI stored in the browser memory. The result is that when you ‘hide’ a DOM element (like a tab) in one of these frameworks; it doesn’t add a display:none;
style attribute, but instead it literally disappears from the ‘real’ DOM.
This allows us to write more declarative code that is cleaner and easier to read. But this is at a cost. Typically, the bundle size of these frameworks is large and for those coming from a jQuery background, the learning curve feels incredibly steep. Especially when all you want to do is toggle tabs! And that is where Alpine.js steps in.
Like Vue and React, Alpine.js allows us to write declarative code but it uses the “real” DOM; amending the contents and attributes of the same nodes that you and I might edit when we crack open a text editor or dev-tools. As a result, you can lose the filesize, wizardry and cognitive-load of larger framework but retain the declarative programming methodology. And you get this with no bundler, no build process and no script tag. Just load 6kb of Alpine.js and you’re away!
Alpine.js | JQuery | Vue.js | React + React DOM | |
---|---|---|---|---|
Coding style | Declarative | Imperative | Declarative | Declarative |
Requires bundler | No | No | No | Yes |
Filesize (GZipped, minified) | 6.4kb | 30kb | 32kb | 5kb + 36kb |
Dev-Tools | No | No | Yes | Yes |
When Should I Reach For Alpine?
For me, Alpine’s strength is in the ease of DOM manipulation. Think of those things you used out of the box with Bootstrap, Alpine.js is great for them. Examples would be:
- Showing and hiding DOM nodes under certain conditions,
- Binding user input,
- Listening for events and altering the UI accordingly,
- Appending classes.
You can also use Alpine.js for templating if your data is available in JSON, but let’s save that for another day.
When Should I Look Elsewhere?
If you’re fetching data, or need to carry out additional functions like validation or storing data, you should probably look elsewhere. Larger frameworks also come with dev-tools which can be invaluable when building larger UIs.
From jQuery To Vue To Alpine
Two years ago, Sarah Drasner posted an article on Smashing Magazine, “Replacing jQuery With Vue.js: No Build Step Necessary,” about how Vue could replace jQuery for many projects. That article started me on a journey which led me to use Vue almost every time I build a user interface. Today, we are going to recreate some of her examples with Alpine, which should illustrate its advantages over both jQuery and Vue in certain use cases.
Alpine’s syntax is almost entirely lifted from Vue.js. In total, there are 13 directives. We’ll cover most of them in the following examples.
Getting Started
Like Vue and jQuery, no build process is required. Unlike Vue, Alpine it initializes itself, so there’s no need to create a new instance. Just load Alpine and you’re good to go.
<script src="https://cdn.jsdelivr.net/gh/alpinejs/alpine@v1.9.4/dist/alpine.js" defer></script>
The scope of any given component is declared using the x-data
directive. This kicks things off and sets some default values if required:
<div x-data="{ foo: 'bar' }">...</div>
Capturing User Inputs
x-model
allow us to keep any input element in sync with the values set using x-data
. In the following example, we set the name value to an empty string (within the form
tag). Using x-model
, we bind this value to the input field. By using x-text
, we inject the value into the innerText
of the paragraph element.
See the Pen Capturing user input with Alpine.js by Phil on CodePen.
This highlights the key differences with Alpine.js and both jQuery and Vue.js.
Updating the paragraph tag in jQuery would require us to listen for specific events (keyup?), explicitly identify the node we wish to update and the changes we wish to make. Alpine’s syntax on the other hand, just specifies what should happen. This is what is meant by declarative programming.
Updating the paragraph in Vue while simple, would require a new script tag:
new Vue({ el: '#app', data: { name: '' } });
While this might not seem like the end of the world, it highlights the first major gain with Alpine. There is no context-switching. Everything is done right there in the HTML — no need for any additional JavaScript.
Click Events, Boolean Attributes And Toggling Classes
Like with Vue, :
serves as a shorthand for x-bind
(which binds attributes) and @
is shorthand for x-on
(which indicates that Alpine should listen for events).
In the following example, we instantiate a new component using x-data
, and set the default value of show
to be false. When the button is clicked, we toggle the value of show
. When this value is true, we instruct Alpine to append the aria-expanded
attribute.
x-bind
works differently for classes: we pass in object where the key is the class-name (active
in our case) and the value is a boolean expression (show
).
See the Pen Click Events, Boolean Attributes and Toggling Classes with Alpine.js by Phil on CodePen.
Hiding And Showing
The syntax showing and hiding is almost identical to Vue.
See the Pen Showing / hiding with Alpine.js by Phil on CodePen.
This will set a given DOM node to display:none
. If you need to remove a DOM element completely, x-if
can be used. However, because Alpine.js doesn’t use the Virtual DOM, x-if
can only be used on a <template></template>
(tag that wraps the element you wish to hide).
Magic Properties
In addition to the above directives, three Magic Properties provide some additional functionality. All of these will be familiar to anyone working in Vue.js.
$el
fetches the root component (the thing with the x-data attribute);$refs
allows you to grab a DOM element;$nextTick
ensures expressions are only executed once Alpine has done its thing;$event
can be used to capture a nature browser event.
See the Pen Magic Properties by Phil on CodePen.
Let’s Build Something Useful
It’s time to build something for the real world. In the interests of brevity I’m going to use Bootstrap for styles, but use Alpine.js for all the JavaScript. The page we’re building is a simple landing page with a contact form displayed inside a modal that submits to some form handler and displays a nice success message. Just the sort of thing a client might ask for and expect pronto!
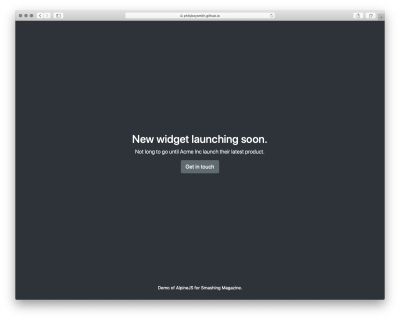
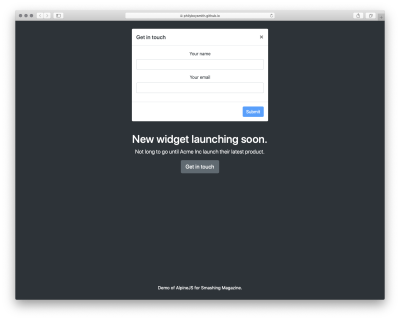
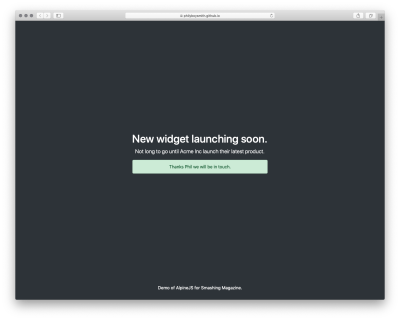
Note: You can view the original markup here.
To make this work, we could add jQuery and Bootstrap.js, but that is quite a bit of overhead for not a lot of functionality. We could probably write it in Vanilla JS, but who wants to do that? Let’s make it work with Alpine.js instead.
First, let’s set a scope and some initial values:
<body class="text-center text-white bg-dark h-100 d-flex flex-column" x-data="{ showModal: false, name: '', email: '', success: false }">
Now, let’s make our button set the showModal
value to true:
<button class="btn btn-lg btn-secondary" @click="showModal = true" >Get in touch</button>
When showModal
is true, we need to display the modal and add some classes:
<div class="modal fade text-dark" :class="{ 'show d-block': showModal }" x-show="showModal" role="dialog">
Let’s bind the input values to Alpine:
<input type="text" class="form-control" name="name" x-model="name" >
<input type="email" class="form-control" name="email" x-model="email" >
And disable the ‘Submit’ button, until those values are set:
<button type="button" class="btn btn-primary" :disabled="!name || !email">Submit</button>
Finally, let’s send data to some kind of asynchronous function, and hide the modal when we’re done:
<button type="button" class="btn btn-primary" :disabled="!name || !email" @click="submitForm({name: name, email: email}).then(() => {showModal = false; success= true;})">Submit</button>
And that’s about it!
See the Pen Something useful built with Alpine.js by Phil on CodePen.
Just Enough JavaScript
When building websites, I’m increasingly trying to ask myself what would be “just enough JavaScript”? When building a sophisticated web application, that might well be React. But when building a marketing site, or something similar, Alpine.js feels like enough. (And even if it’s not, given the similar syntax, switching to Vue.js takes no time at all).
It’s incredibly easy to use (especially if you’ve never used VueJS). It’s tiny (< 6kb gzipped). And it means no more context switching between HTML and JavaScript files.
There are more advanced features that aren’t included in this article and Caleb is constantly adding new features. If you want to find out more, take a look at the official docs on Github.
Further Reading
- The Era Of Platform Primitives Is Finally Here
- Recovering Deleted Files From Your Git Working Tree
- How To Build Server-Side Rendered (SSR) Svelte Apps With SvelteKit
- Building A Retro Draggable Web Component With Lit
