How To Build Server-Side Rendered (SSR) Svelte Apps With SvelteKit
I’m not interested in starting a turf war between server-side rendering and client-side rendering. The fact is that SvelteKit supports both, which is one of the many perks it offers right out of the box. The server-side rendering paradigm is not a new concept. It means that the client (i.e., the user’s browser) sends a request to the server, and the server responds with the data and markup for that particular page, which is then rendered in the user’s browser.
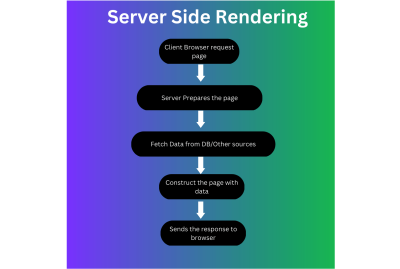
To build an SSR app using the primary Svelte framework, you would need to maintain two codebases, one with the server running in Node, along with with some templating engine, like Handlebars or Mustache. The other application is a client-side Svelte app that fetches data from the server.
The approach we’re looking at in the above paragraph isn’t without disadvantages. Two that immediately come to mind that I’m sure you thought of after reading that last paragraph:
- The application is more complex because we’re effectively maintaining two systems.
- Sharing logic and data between the client and server code is more difficult than fetching data from an API on the client side.
SvelteKit Simplifies The Process
SvelteKit streamlines things by handling of complexity of the server and client on its own, allowing you to focus squarely on developing the app. There’s no need to maintain two applications or do a tightrope walk sharing data between the two.
Here’s how:
- Each route can have a
page.server.ts
file that’s used to run code in the server and return data seamlessly to your client code. - If you use TypeScript, SvelteKit auto-generates types that are shared between the client and server.
- SvelteKit provides an option to select your rendering approach based on the route. You can choose SSR for some routes and CSR for others, like maybe your admin page routes.
- SvelteKit also supports routing based on a file system, making it much easier to define new routes than having to hand-roll them yourself.
SvelteKit In Action: Job Board
I want to show you how streamlined the SvelteKit approach is to the traditional way we have been dancing between the SSR and CSR worlds, and I think there’s no better way to do that than using a real-world example. So, what we’re going to do is build a job board — basically a list of job items — while detailing SvelteKit’s role in the application.
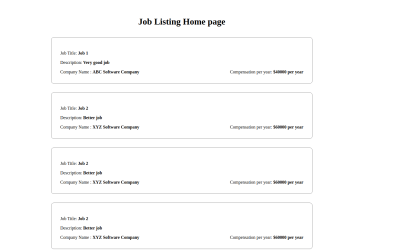
When we’re done, what we’ll have is an app where SvelteKit fetches the data from a JSON file and renders it on the server side. We’ll go step by step.
First, Initialize The SvelteKit Project
The official SvelteKit docs already do a great job of explaining how to set up a new project. But, in general, we start any SvelteKit project in the command line with this command:
npm create svelte@latest job-list-ssr-sveltekit
This command creates a new project folder called job-list-ssr-sveltekit
on your machine and initializes Svelte and SvelteKit for us to use. But we don’t stop there — we get prompted with a few options to configure the project:
- First, we select a SvelteKit template. We are going to stick to using the basic Skeleton Project template.
- Next, we can enable type-checking if you’re into that. Type-checking provides assistance when writing code by watching for bugs in the app’s data types. I’m going to use the “TypeScript syntax” option, but you aren’t required to use it and can choose the “None” option instead.
There are additional options from there that are more a matter of personal preference:
- ESLint to enforce code consistency,
- Prettier to clean up code formatting,
- Playwright for browser testing locally,
- Vitest for unit testing.
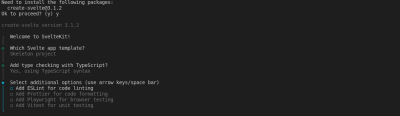
If you are familiar with any of these, you can add them to the project. We are going to keep it simple and not select anything from the list since what I really want to show off is the app architecture and how everything works together to get data rendered by the app.
Now that we have the template for our project ready for us let’s do the last bit of setup by installing the dependencies for Svelte and SvelteKit to do their thing:
cd job-listing-ssr-sveltekit
npm install
There’s something interesting going on under the hood that I think is worth calling out:
Is SvelteKit A Dependency?
If you are new to Svelte or SvelteKit, you may be pleasantly surprised when you open the project’s package.json
file. Notice that the SvelteKit is listed in the devDependencies
section. The reason for that is Svelte (and, in turn, SvelteKit) acts like a compiler that takes all your .js
and .svelte
files and converts them into optimized JavaScript code that is rendered in the browser.
This means the Svelte package is actually unnecessary when we deploy it to the server. That’s why it is not listed as a dependency in the package file. The final bundle of our job board app is going to contain just the app’s code, which means the size of the bundle is way smaller and loads faster than the regular Svelte-based architecture.
Look at how tiny and readable the package-json
file is!
{
"name": "job-listing-ssr-sveltekit",
"version": "0.0.1",
"private": true,
"scripts": {
"dev": "vite dev",
"build": "vite build",
"preview": "vite preview",
"check": "svelte-kit sync && svelte-check --tsconfig ./tsconfig.json",
"check:watch": "svelte-kit sync && svelte-check --tsconfig ./tsconfig.json --watch"
},
"devDependencies": {
"@sveltejs/adapter-auto": "^2.0.0",
"@sveltejs/kit": "^1.5.0",
"svelte": "^3.54.0",
"svelte-check": "^3.0.1",
"tslib": "^2.4.1",
"typescript": "^4.9.3",
"vite": "^4.0.0"
},
"type": "module"
}
I really find this refreshing, and I hope you do, too. Seeing a big list of packages tends to make me nervous because all those moving pieces make the entirety of the app architecture feel brittle and vulnerable. The concise SvelteKit output, by contrast, gives me much more confidence.
Creating The Data
We need data coming from somewhere that can inform the app on what needs to be rendered. I mentioned earlier that we would be placing data in and pulling it from a JSON file. That’s still the plan.
As far as the structured data goes, what we need to define are properties for a job board item. Depending on your exact needs, there could be a lot of fields or just a few. I’m going to proceed with the following:
- Job title,
- Job description,
- Company Name,
- Compensation.
Here’s how that looks in JSON:
[{
"job_title": "Job 1",
"job_description": "Very good job",
"company_name": "ABC Software Company",
"compensation_per_year": "$40000 per year"
}, {
"job_title": "Job 2",
"job_description": "Better job",
"company_name": "XYZ Software Company",
"compensation_per_year": "$60000 per year"
}]
Now that we’ve defined some data let’s open up the main project folder. There’s a sub-directory in there called src
. We can open that and create a new folder called data
and add the JSON file we just made to it. We will come back to the JSON file when we work on fetching the data for the job board.
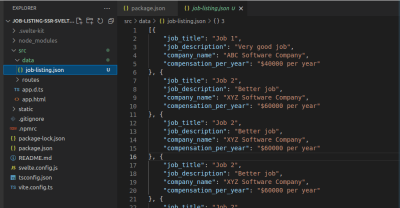
Adding TypeScript Model
Again, TypeScript is completely optional. But since it’s so widely used, I figure it’s worth showing how to set it up in a SvelteKit framework.
We start by creating a new models.ts
file in the project’s src
folder. This is the file where we define all of the data types that can be imported and used by other components and pages, and TypeScript will check them for us.
Here’s the code for the models.ts
file:
export type JobsList = JobItem[]
export interface JobItem {
job_title: string
job_description: string
company_name: string
compensation_per_year: string
}
There are two data types defined in the code:
JobList
contains the array of job items.JobItem
contains the job details (or properties) that we defined earlier.
The Main Job Board Page
We’ll start by developing the code for the main job board page that renders a list of available job items. Open the src/routes/+page.svelte
file, which is the main job board. Notice how it exists in the /src/routes
folder? That’s the file-based routing system I referred to earlier when talking about the benefits of SvelteKit. The name of the file is automatically generated into a route. That’s a real DX gem, as it saves us time from having to code the routes ourselves and maintaining more code.
While +page.svelte
is indeed the main page of the app, it’s also the template for any generic page in the app. But we can create a separation of concerns by adding more structure in the /scr/routes
directory with more folders and sub-folders that result in different paths. SvelteKit’s docs have all the information you need for routing and routing conventions.
This is the markup and styles we’ll use for the main job board:
<div class="home-page">
<h1>Job Listing Home page</h1>
</div>
<style>
.home-page {
padding: 2rem 4rem;
display: flex;
align-items: center;
flex-direction: column;
justify-content: center;
}
</style>
Yep, this is super simple. All we’re adding to the page is an <h1>
tag for the page title and some light CSS styling to make sure the content is centered and has some nice padding for legibility. I don’t want to muddy the waters of this example with a bunch of opinionated markup and styles that would otherwise be a distraction from the app architecture.
Run The App
We’re at a point now where we can run the app using the following in the command line:
npm run dev -- --open
The -- --open
argument automatically opens the job board page in the browser. That’s just a small but nice convenience. You can also navigate to the URL that the command line outputs.
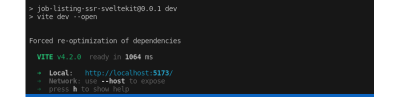
The Job Item Component
OK, so we have a main job board page that will be used to list job items from the data fetched by the app. What we need is a new component specifically for the jobs themselves. Otherwise, all we have is a bunch of data with no instructions for how it is rendered.
Let’s take of that by opening the src
folder in the project and creating a new sub-folder called components
. And in that new /src/components
folder, let’s add a new Svelte file called JobDisplay.svelte
.
We can use this for the component’s markup and styles:
<script lang="ts">
import type { JobItem } from "../models";
export let job: JobItem;
</script>
<div class="job-item">
<p>Job Title: <b>{job.job_title}</b></p>
<p>Description: <b>{job.job_description}</b></p>
<div class="job-details">
<span>Company Name : <b>{job.company_name}</b></span>
<span>Compensation per year: <b>{job.compensation_per_year}</b></span>
</div>
</div>
<style>
.job-item {
border: 1px solid grey;
padding: 2rem;
width: 50%;
margin: 1rem;
border-radius: 10px;
}
.job-details {
display: flex;
justify-content: space-between;
}
</style>
Let’s break that down so we know what’s happening:
- At the top, we import the TypeScript
JobItem
model. - Then, we define a
job
prop with a type ofJobItem
. This prop is responsible for getting the data from its parent component so that we can pass that data to this component for rendering. - Next, the HTML provides this component’s markup.
- Last is the CSS for some light styling. Again, I’m keeping this super simple with nothing but a little padding and minor details for structure and legibility. For example,
justify-content: space-between
adds a little visual separation between job items.
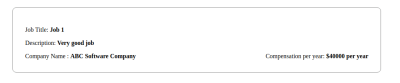
Fetching Job Data
Now that we have the JobDisplay
component all done, we’re ready to pass it data to fill in all those fields to be displayed in each JobDisplay
rendered on the main job board.
Since this is an SSR application, the data needs to be fetched on the server side. SvelteKit makes this easy by having a separate load
function that can be used to fetch data and used as a hook for other actions on the server when the page loads.
To fetch, let’s create yet another new file TypeScript file — this time called +page.server.ts
— in the project’s routes
directory. Like the +page.svelte
file, this also has a special meaning which will make this file run in the server when the route is loaded. Since we want this on the main job board page, we will create this file in the routes
directory and include this code in it:
import jobs from ’../data/job-listing.json’
import type { JobsList } from ’../models’;
const job_list: JobsList = jobs;
export const load = (() => {
return {
job_list
};
})
Here’s what we’re doing with this code:
- We import data from the JSON file. This is for simplicity purposes. In the real app, you would likely fetch this data from a database by making an API call.
- Then, we import the TypeScript model we created for
JobsList
. - Next, we create a new
job_list
variable and assign the imported data to it. - Last, we define a
load
function that will return an object with the assigned data. SvelteKit will automatically call this function when the page is requested. So, the magic for SSR code happens here as we fetch the data in the server and build the HTML with the data we get back.
Accessing Data From The Job Board
SvelteKit makes accessing data relatively easy by passing data to the main job board page in a way that checks the types for errors in the process. We can import a type called PageServerData
in the +page.svelte
file. This type is autogenerated and will have the data returned by the +page.server.ts
file. This is awesome, as we don’t have to define types again when using the data we receive.
Let’s update the code in the +page.svelte
file, like the following:
<script lang="ts">
import JobDisplay from ’../components/JobDisplay.svelte’;
import type { PageServerData } from ’./$types’;
export let data: PageServerData;
</script>
<div class="home-page">
<h1>Job Listing Home page</h1>
{#each data.job_list as job}
<JobDisplay job={job}/>
{/each}
</div>
<style>....</style>
This is so cool because:
- The
#each
syntax is a Svelte benefit that can be used to repeat theJobDisplay
component for all the jobs for which data exists. - At the top, we are importing both the JobDisplay component and
PageServerData
type from./$types
, which is autogenerated by SvelteKit.
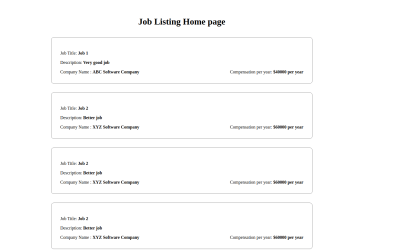
Deploying The App
We’re ready to compile and bundle this project in preparation for deployment! We get to use the same command in the Terminal as most other frameworks, so it should be pretty familiar:
npm run build
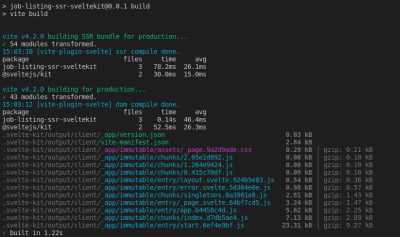
Note: You might get the following warning when running that command: “Could not detect a supported production environment.” We will fix that in just a moment, so stay with me.
From here, we can use the npm run preview
command to check the latest built version of the app:
npm run preview
This process is a new way to gain confidence in the build locally before deploying it to a production environment.
The next step is to deploy the app to the server. I’m using Netlify, but that’s purely for example, so feel free to go with another option. SvelteKit offers adapters that will deploy the app to different server environments. You can get the whole list of adapters in the docs, of course.
The real reason I’m using Netlify is that deploying there is super convenient for this tutorial, thanks to the adapter-netlify
plugin that can be installed with this command:
npm i -D @sveltejs/adapter-netlify
This does, indeed, introduce a new dependency in the package.json
file. I mention that because you know how much I like to keep that list short.
After installation, we can update the svelte.config.js
file to consume the adapter:
import adapter from ’@sveltejs/adapter-netlify’;
import { vitePreprocess } from ’@sveltejs/kit/vite’;
/** @type {import(’@sveltejs/kit’).Config} */
const config = {
preprocess: vitePreprocess(),
kit: {
adapter: adapter({
edge: false,
split: false
})
}
};
export default config;
Real quick, this is what’s happening:
- The
adapter
is imported fromadapter-netlify
. - The new adapter is passed to the
adapter
property inside thekit
. - The
edge
boolean value can be used to configure the deployment to a Netlify edge function. - The
split
boolean value is used to control whether we want to split each route into separate edge functions.
More Netlify-Specific Configurations
Everything from here on out is specific to Netlify, so I wanted to break it out into its own section to keep things clear.
We can add a new file called netlify.toml
at the top level of the project folder and add the following code:
[build]
command = "npm run build"
publish = "build"
I bet you know what this is doing, but now we have a new alias for deploying the app to Netlify. It also allows us to control deployment from a Netlify account as well, which might be a benefit to you. To do this, we have to:
- Create a new project in Netlify,
- Select the “Import an existing project” option, and
- Provide permission for Netlify to access the project repository. You get to choose where you want to store your repo, whether it’s GitHub or some other service.
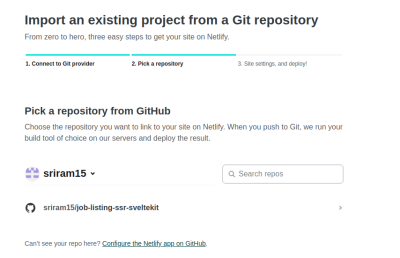
Since we have set up the netlify.toml
file, we can leave the default configuration and click the “Deploy” button directly from Netlify.
Once the deployment is completed, you can navigate to the site using the provided URL in Netlify. This should be the final result:
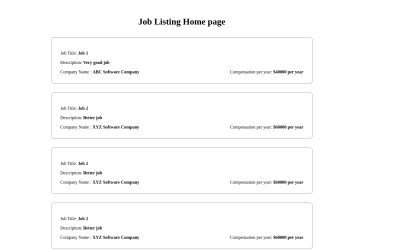
Here’s something fun. Open up DevTools when viewing the app in the browser and notice that the HTML contains the actual data we fetched from the JSON file. This way, we know for sure that the right data is rendered and that everything is working.
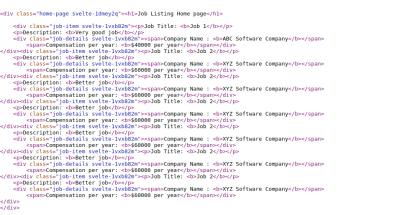
Note: The source code of the whole project is available on GitHub. All the steps we covered in this article are divided as separate commits in the main
branch for your reference.
Conclusion
In this article, we have learned about the basics of server-side rendered apps and the steps to create and deploy a real-life app using SvelteKit as the framework. Feel free to share your comments and perspective on this topic, especially if you are considering picking SvelteKit for your next project.
Further Reading On SmashingMag
- Differences Between Static Generated Sites And Server-Side Rendered Apps, Timi Omoyeni
- How To Use Google CrUX To Analyze And Compare The Performance Of JS Frameworks, Dan Shappir
- A Look At Remix And The Differences With Next.js, Facundo Giuliani
- Building A Server-Side Application With Async Functions and Koa 2
