How To Use Artificial Intelligence And Machine Learning To Summarize Chat Conversations
As developers, we often deal with large volumes of text, and making sense of it can be a challenge. In many cases, we might only be interested in a summary of the text or a quick overview of its main points. This is where text summarization comes in.
Text summarization is the process of automatically creating a shorter version of a text that preserves its key information. It has many applications in natural language processing (NLP), from summarizing news articles to generating abstracts for scientific papers. Even products, including Notion, are integrating AI features that will summarize a block of text on command.
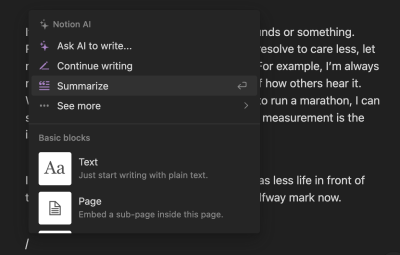
One interesting use case is summarizing chat conversations, where the goal is to distill the main topics and ideas discussed during the conversation. That’s what we are going to explore in this article. Whether you’re an experienced developer or just getting started with natural language processing, this article will provide a practical guide to building a chat summarizer from scratch. By the end, you’ll have a working chat summarizer that you can use to extract the main ideas from your own chat conversations — or any other text data that you might encounter in your projects.
The best part about all of this is that accessing and integrating these sorts of AI and NLP capabilities is easier than ever. Where something like this may have required workarounds and lots of dependencies in the not-so-distant past, there are APIs and existing models readily available that we can leverage. I think you may even be surprised by how few steps there are to pull off this demo of a tool that summarizes chat conversations.
Cohere: Chat Summarization Made Easy
Cohere is a cloud-based natural language processing platform that enables developers to build sophisticated language models without requiring deep expertise in machine learning. It offers a range of powerful tools for text classification, entity extraction, sentiment analysis, and more. One of its most popular features is chat summarization, which can automatically generate a summary of a conversation.
Using Cohere API for chat summarization is a simple and effective way to summarize chat conversations. It requires only a few lines of code to be implemented and can be used to summarize any chat conversation in real-time.
The chat summarization function of Cohere works by using natural language processing algorithms to analyze the text of the conversation. These algorithms identify important sentences and phrases, along with contextual information like speaker identity, timestamps, and sentiment. The output is a brief summary of the conversation that includes essential information and main points.
Using The Cohere API For Chat Summarization
Now that we have a basic understanding of Cohere API and its capabilities, let’s dive into how we can use it to generate chat summaries. In this section, we will discuss the step-by-step process of generating chat summaries using Cohere API.
To get started with the Cohere API, first, you’ll need to sign up for an API key on the Cohere website. Once you have an API key, you can install the Cohere Python package using pip:
pip install cohere
Next, you’ll need to initialize the cohere client by providing the API key:
import cohere
# initialize Cohere client
co = cohere.Client("YOUR_API_KEY")
Once the client is initialized, we can provide input for the summary. In the case of chat summarization, we need to provide the conversation as input. Here’s how you can provide input for the summary:
conversation = """
Senior Dev: Hey, have you seen the latest pull request for the authentication module?
Junior Dev: No, not yet. What’s in it?
Senior Dev: They’ve added support for JWT tokens, so we can use that instead of session cookies for authentication.
Junior Dev: Oh, that’s great. I’ve been wanting to switch to JWT for a while now.
Senior Dev: Yeah, it’s definitely more secure and scalable. I’ve reviewed the code and it looks good, so go ahead and merge it if you’re comfortable with it.
Junior Dev: Will do, thanks for the heads-up!
"""
Now that we provided the input, we can generate the summary using the co.summarize() method. We can also specify the parameters for the summary, such as the model, length, and extractiveness ( . Here’s how you can generate the summary:
response = co.summarize(conversation, model = 'summarize-xlarge', length = 'short', extractiveness = 'high', temperature = 0.5,)summary = response.summary
Finally, we can output the summary using print() or any other method of our choice. Here’s how you can output the summary
print(summary)
And that’s it! With these simple steps, we can generate chat summaries using Cohere API. In the next section, we will discuss how we can deploy the chat summarizer using Gradio.
Deploying The Chat Summarizer To Gradio
Gradio is a user interface library for quickly prototyping machine learning (ML) models. By deploying our chat summarizer model in Gradio, we can create a simple and intuitive interface that anyone can use to summarize conversations.
To get started, we need to import the necessary libraries:
import gradio as gr
import cohere
If you don’t have Gradio installed on your machine yet, don’t worry! You can easily install it using pip. Open up your terminal or command prompt and enter the following command:
!pip install gradio
This will install the latest version of Gradio and any dependencies that it requires. Once you’ve installed Gradio, you’re ready to start building your own machine learning-powered user interfaces.
Next, we need to initialize the Cohere client. This is done using the following line of code:
co = cohere.Client("YOUR API KEY")
The Client object allows us to interact with the CoHere API, and the API key is passed as an argument to authenticate the client.Now we can define the chat summarizer function:
def chat_summarizer(conversation):
# generate summary using Cohere API
response = co.summarize(conversation, model = 'summarize-xlarge', length = 'short', extractiveness = 'high', temperature = 0.5)
summary = response.summary
return summary
The chat_summarizer function takes the conversation text as input and generates a summary using the Cohere API. We pass the conversation text to the co.summarize method, along with the parameters that specify the model to use and the length and extractiveness of the summary.
Finally, we can create the Gradio interface using the following code:
chat_input = gr.inputs.Textbox(lines = 10, label = "Conversation")
chat_output = gr.outputs.Textbox(label = "Summary")
chat_interface = gr.Interface(
fn = chat_summarizer,
inputs = chat_input,
outputs = chat_output,
title = "Chat Summarizer",
description = "This app generates a summary of a chat conversation using Cohere API."
)
The gr.inputs.textbox
and gr.outputs.textbox
objects define the input and output fields of the interface, respectively. We pass these objects, along with the chat_summarizer
function, to the gr.Interface
constructor to create the interface. We also provide a title and description for the interface.
To launch the interface, we call the launch method on the interface object:
chat_interface.launch()
This will launch a webpage with our interface where users can enter their dialogue and generate a summary with a single click.
Conclusion
In today’s fast-paced digital world, where communication happens mostly through chat, chat summarization plays a vital role in saving time and improving productivity. The ability to quickly and accurately summarize lengthy chat conversations can help individuals and businesses make informed decisions and avoid misunderstandings.
Imagine using it to summarize a chain of email replies, saving you time from having to untangle the conversation yourself. Or perhaps you’re reviewing a particularly dense webpage of content, and the summarizer can help distill the essential points.
With the help of advanced AI and NLP techniques, summarization features have become more accurate and efficient than ever before. So, if you haven’t tried summarizing yet, I highly encourage you to give it a try and share your feedback. It could be a game-changer in your daily communication routine.
